Note
Click here to download the full example code
Coordinate-based meta-analysis algorithms
A tour of CBMA algorithms in NiMARE.
This tutorial is intended to provide a brief description and example of each of the CBMA algorithms implemented in NiMARE. For a more detailed introduction to the elements of a coordinate-based meta-analysis, see other stuff.
Load Dataset
Note
The data used in this example come from a collection of NIDM-Results packs downloaded from Neurovault collection 1425, uploaded by Dr. Camille Maumet.
Creation of the Dataset from the NIDM-Results packs was done with custom code. The Results packs for collection 1425 are not completely NIDM-Results-compliant, so the nidmresults library could not be used to facilitate data extraction.
import os
from nilearn.plotting import plot_stat_map
from nimare.correct import FWECorrector
from nimare.dataset import Dataset
from nimare.utils import get_resource_path
dset_file = os.path.join(get_resource_path(), "nidm_pain_dset.json")
dset = Dataset(dset_file)
# Some of the CBMA algorithms compare two Datasets,
# so we'll split this example Dataset in half.
dset1 = dset.slice(dset.ids[:10])
dset2 = dset.slice(dset.ids[10:])
Multilevel Kernel Density Analysis
from nimare.meta.cbma.mkda import MKDADensity
meta = MKDADensity()
results = meta.fit(dset)
corr = FWECorrector(method="montecarlo", n_iters=10, n_cores=1)
cres = corr.transform(results)
plot_stat_map(
results.get_map("z"),
cut_coords=[0, 0, -8],
draw_cross=False,
cmap="RdBu_r",
threshold=0.1,
)
plot_stat_map(
cres.get_map("z_level-voxel_corr-FWE_method-montecarlo"),
cut_coords=[0, 0, -8],
draw_cross=False,
cmap="RdBu_r",
threshold=0.1,
)
Out:
0%| | 0/10 [00:00<?, ?it/s]
10%|# | 1/10 [00:00<00:03, 2.43it/s]
20%|## | 2/10 [00:00<00:03, 2.56it/s]
30%|### | 3/10 [00:01<00:02, 2.60it/s]
40%|#### | 4/10 [00:01<00:02, 2.62it/s]
50%|##### | 5/10 [00:01<00:01, 2.62it/s]
60%|###### | 6/10 [00:02<00:01, 2.61it/s]
70%|####### | 7/10 [00:02<00:01, 2.62it/s]
80%|######## | 8/10 [00:03<00:00, 2.62it/s]
90%|######### | 9/10 [00:03<00:00, 2.63it/s]
100%|##########| 10/10 [00:03<00:00, 2.64it/s]
100%|##########| 10/10 [00:03<00:00, 2.62it/s]
<nilearn.plotting.displays._slicers.OrthoSlicer object at 0x7f183abcfa90>
MKDA Chi-Squared
from nimare.meta.cbma.mkda import MKDAChi2
meta = MKDAChi2(kernel__r=10)
results = meta.fit(dset1, dset2)
corr = FWECorrector(method="montecarlo", n_iters=10, n_cores=1)
cres = corr.transform(results)
plot_stat_map(
results.get_map("z_desc-consistency"),
draw_cross=False,
cmap="RdBu_r",
threshold=0.1,
)
plot_stat_map(
cres.get_map("z_desc-consistencySize_level-cluster_corr-FWE_method-montecarlo"),
draw_cross=False,
cmap="RdBu_r",
threshold=0.1,
)
Out:
/home/docs/checkouts/readthedocs.org/user_builds/nimare/checkouts/0.0.12rc7/nimare/meta/cbma/mkda.py:368: RuntimeWarning: invalid value encountered in true_divide
pFgA = pAgF * pF / pA
/home/docs/checkouts/readthedocs.org/user_builds/nimare/checkouts/0.0.12rc7/nimare/meta/cbma/mkda.py:374: RuntimeWarning: invalid value encountered in true_divide
pFgA_prior = pAgF * self.prior / pAgF_prior
0%| | 0/10 [00:00<?, ?it/s]
10%|# | 1/10 [00:00<00:05, 1.55it/s]
20%|## | 2/10 [00:01<00:04, 1.60it/s]
30%|### | 3/10 [00:01<00:04, 1.62it/s]
40%|#### | 4/10 [00:02<00:03, 1.63it/s]
50%|##### | 5/10 [00:03<00:03, 1.64it/s]
60%|###### | 6/10 [00:03<00:02, 1.63it/s]
70%|####### | 7/10 [00:04<00:01, 1.64it/s]
80%|######## | 8/10 [00:04<00:01, 1.65it/s]
90%|######### | 9/10 [00:05<00:00, 1.65it/s]
100%|##########| 10/10 [00:06<00:00, 1.65it/s]
100%|##########| 10/10 [00:06<00:00, 1.64it/s]
<nilearn.plotting.displays._slicers.OrthoSlicer object at 0x7f1848e19690>
Kernel Density Analysis
from nimare.meta.cbma.mkda import KDA
meta = KDA()
results = meta.fit(dset)
corr = FWECorrector(method="montecarlo", n_iters=10, n_cores=1)
cres = corr.transform(results)
plot_stat_map(
results.get_map("z"),
cut_coords=[0, 0, -8],
draw_cross=False,
cmap="RdBu_r",
threshold=0.1,
)
plot_stat_map(
cres.get_map("z_desc-size_level-cluster_corr-FWE_method-montecarlo"),
cut_coords=[0, 0, -8],
draw_cross=False,
cmap="RdBu_r",
threshold=0.1,
)
Out:
0%| | 0/10 [00:00<?, ?it/s]/home/docs/checkouts/readthedocs.org/user_builds/nimare/envs/0.0.12rc7/lib/python3.7/site-packages/nilearn/image/image.py:756: FutureWarning: Image data has type int64, which may cause incompatibilities with other tools. This will error in NiBabel 5.0. This warning can be silenced by passing the dtype argument to Nifti1Image().
return klass(data, affine, header=header)
10%|# | 1/10 [00:00<00:03, 2.97it/s]
20%|## | 2/10 [00:00<00:02, 2.98it/s]
30%|### | 3/10 [00:00<00:02, 3.04it/s]
40%|#### | 4/10 [00:01<00:01, 3.06it/s]
50%|##### | 5/10 [00:01<00:01, 3.05it/s]
60%|###### | 6/10 [00:01<00:01, 3.05it/s]
70%|####### | 7/10 [00:02<00:00, 3.06it/s]
80%|######## | 8/10 [00:02<00:00, 3.07it/s]
90%|######### | 9/10 [00:02<00:00, 3.06it/s]
100%|##########| 10/10 [00:03<00:00, 3.04it/s]
100%|##########| 10/10 [00:03<00:00, 3.05it/s]
<nilearn.plotting.displays._slicers.OrthoSlicer object at 0x7f183ad51c50>
Activation Likelihood Estimation
from nimare.meta.cbma.ale import ALE
meta = ALE()
results = meta.fit(dset)
corr = FWECorrector(method="montecarlo", n_iters=10, n_cores=1)
cres = corr.transform(results)
plot_stat_map(
results.get_map("z"),
cut_coords=[0, 0, -8],
draw_cross=False,
cmap="RdBu_r",
threshold=0.1,
)
plot_stat_map(
cres.get_map("z_desc-size_level-cluster_corr-FWE_method-montecarlo"),
cut_coords=[0, 0, -8],
draw_cross=False,
cmap="RdBu_r",
threshold=0.1,
)
Out:
0%| | 0/10 [00:00<?, ?it/s]
10%|# | 1/10 [00:00<00:03, 2.64it/s]
20%|## | 2/10 [00:00<00:02, 2.71it/s]
30%|### | 3/10 [00:01<00:02, 2.75it/s]
40%|#### | 4/10 [00:01<00:02, 2.75it/s]
50%|##### | 5/10 [00:01<00:01, 2.74it/s]
60%|###### | 6/10 [00:02<00:01, 2.73it/s]
70%|####### | 7/10 [00:02<00:01, 2.73it/s]
80%|######## | 8/10 [00:02<00:00, 2.73it/s]
90%|######### | 9/10 [00:03<00:00, 2.73it/s]
100%|##########| 10/10 [00:03<00:00, 2.74it/s]
100%|##########| 10/10 [00:03<00:00, 2.73it/s]
<nilearn.plotting.displays._slicers.OrthoSlicer object at 0x7f1848de9150>
Specific Co-Activation Likelihood Estimation
Important
The SCALE algorithm is very memory intensive, so we don’t run it within the documentation.
ALE-Based Subtraction Analysis
from nimare.meta.cbma.ale import ALESubtraction
meta = ALESubtraction(n_iters=10, n_cores=1)
results = meta.fit(dset1, dset2)
plot_stat_map(
results.get_map("z_desc-group1MinusGroup2"),
cut_coords=[0, 0, -8],
draw_cross=False,
cmap="RdBu_r",
threshold=0.1,
)
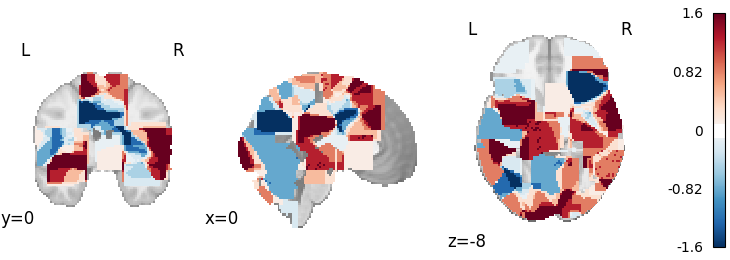
Out:
0%| | 0/10 [00:00<?, ?it/s]
60%|###### | 6/10 [00:00<00:00, 53.09it/s]
100%|##########| 10/10 [00:00<00:00, 53.03it/s]
0%| | 0/228483 [00:00<?, ?it/s]
0%| | 1024/228483 [00:00<00:22, 10233.40it/s]
1%| | 2068/228483 [00:00<00:21, 10353.96it/s]
1%|1 | 3109/228483 [00:00<00:21, 10376.33it/s]
2%|1 | 4160/228483 [00:00<00:21, 10428.17it/s]
2%|2 | 5203/228483 [00:00<00:21, 10363.47it/s]
3%|2 | 6253/228483 [00:00<00:21, 10406.42it/s]
3%|3 | 7301/228483 [00:00<00:21, 10429.62it/s]
4%|3 | 8352/228483 [00:00<00:21, 10453.65it/s]
4%|4 | 9398/228483 [00:00<00:21, 10417.80it/s]
5%|4 | 10443/228483 [00:01<00:20, 10425.31it/s]
5%|5 | 11486/228483 [00:01<00:20, 10379.42it/s]
5%|5 | 12531/228483 [00:01<00:20, 10399.75it/s]
6%|5 | 13572/228483 [00:01<00:20, 10395.54it/s]
6%|6 | 14618/228483 [00:01<00:20, 10412.50it/s]
7%|6 | 15660/228483 [00:01<00:20, 10348.22it/s]
7%|7 | 16695/228483 [00:01<00:20, 10320.21it/s]
8%|7 | 17740/228483 [00:01<00:20, 10358.75it/s]
8%|8 | 18776/228483 [00:01<00:20, 10337.78it/s]
9%|8 | 19820/228483 [00:01<00:20, 10367.20it/s]
9%|9 | 20866/228483 [00:02<00:19, 10393.70it/s]
10%|9 | 21906/228483 [00:02<00:19, 10346.91it/s]
10%|# | 22948/228483 [00:02<00:19, 10366.57it/s]
10%|# | 23986/228483 [00:02<00:19, 10368.99it/s]
11%|# | 25031/228483 [00:02<00:19, 10392.70it/s]
11%|#1 | 26071/228483 [00:02<00:19, 10325.32it/s]
12%|#1 | 27113/228483 [00:02<00:19, 10353.21it/s]
12%|#2 | 28155/228483 [00:02<00:19, 10370.59it/s]
13%|#2 | 29193/228483 [00:02<00:27, 7300.16it/s]
13%|#3 | 30226/228483 [00:03<00:24, 8000.36it/s]
14%|#3 | 31258/228483 [00:03<00:22, 8575.83it/s]
14%|#4 | 32301/228483 [00:03<00:21, 9059.66it/s]
15%|#4 | 33339/228483 [00:03<00:20, 9417.77it/s]
15%|#5 | 34393/228483 [00:03<00:19, 9730.07it/s]
15%|#5 | 35405/228483 [00:03<00:19, 9838.20it/s]
16%|#5 | 36439/228483 [00:03<00:19, 9983.34it/s]
16%|#6 | 37477/228483 [00:03<00:18, 10098.73it/s]
17%|#6 | 38515/228483 [00:03<00:18, 10180.46it/s]
17%|#7 | 39563/228483 [00:03<00:18, 10267.49it/s]
18%|#7 | 40597/228483 [00:04<00:18, 10255.50it/s]
18%|#8 | 41646/228483 [00:04<00:18, 10322.65it/s]
19%|#8 | 42686/228483 [00:04<00:17, 10344.50it/s]
19%|#9 | 43727/228483 [00:04<00:17, 10362.44it/s]
20%|#9 | 44765/228483 [00:04<00:17, 10322.99it/s]
20%|## | 45810/228483 [00:04<00:17, 10358.40it/s]
21%|## | 46857/228483 [00:04<00:17, 10388.95it/s]
21%|## | 47897/228483 [00:04<00:17, 10391.06it/s]
21%|##1 | 48937/228483 [00:04<00:17, 10364.88it/s]
22%|##1 | 49983/228483 [00:04<00:17, 10391.85it/s]
22%|##2 | 51023/228483 [00:05<00:17, 10376.59it/s]
23%|##2 | 52061/228483 [00:05<00:17, 10369.15it/s]
23%|##3 | 53099/228483 [00:05<00:17, 10275.41it/s]
24%|##3 | 54137/228483 [00:05<00:16, 10305.20it/s]
24%|##4 | 55187/228483 [00:05<00:16, 10362.35it/s]
25%|##4 | 56224/228483 [00:05<00:16, 10287.82it/s]
25%|##5 | 57263/228483 [00:05<00:16, 10316.92it/s]
26%|##5 | 58300/228483 [00:05<00:16, 10332.12it/s]
26%|##5 | 59342/228483 [00:05<00:16, 10357.76it/s]
26%|##6 | 60389/228483 [00:05<00:16, 10389.87it/s]
27%|##6 | 61429/228483 [00:06<00:16, 10340.40it/s]
27%|##7 | 62476/228483 [00:06<00:15, 10378.06it/s]
28%|##7 | 63515/228483 [00:06<00:15, 10379.81it/s]
28%|##8 | 64554/228483 [00:06<00:15, 10379.54it/s]
29%|##8 | 65592/228483 [00:06<00:15, 10322.40it/s]
29%|##9 | 66625/228483 [00:06<00:15, 10322.17it/s]
30%|##9 | 67658/228483 [00:06<00:22, 7193.54it/s]
30%|### | 68691/228483 [00:06<00:20, 7912.23it/s]
31%|### | 69730/228483 [00:07<00:18, 8522.90it/s]
31%|### | 70757/228483 [00:07<00:17, 8977.06it/s]
31%|###1 | 71805/228483 [00:07<00:16, 9382.63it/s]
32%|###1 | 72846/228483 [00:07<00:16, 9667.72it/s]
32%|###2 | 73893/228483 [00:07<00:15, 9895.27it/s]
33%|###2 | 74916/228483 [00:07<00:15, 9989.79it/s]
33%|###3 | 75940/228483 [00:07<00:15, 10061.14it/s]
34%|###3 | 76991/228483 [00:07<00:14, 10190.64it/s]
34%|###4 | 78024/228483 [00:07<00:14, 10230.29it/s]
35%|###4 | 79072/228483 [00:07<00:14, 10303.80it/s]
35%|###5 | 80120/228483 [00:08<00:14, 10354.79it/s]
36%|###5 | 81160/228483 [00:08<00:14, 10306.25it/s]
36%|###5 | 82202/228483 [00:08<00:14, 10337.90it/s]
36%|###6 | 83238/228483 [00:08<00:14, 10341.01it/s]
37%|###6 | 84285/228483 [00:08<00:13, 10376.75it/s]
37%|###7 | 85324/228483 [00:08<00:13, 10329.65it/s]
38%|###7 | 86365/228483 [00:08<00:13, 10352.37it/s]
38%|###8 | 87406/228483 [00:08<00:13, 10367.16it/s]
39%|###8 | 88450/228483 [00:08<00:13, 10387.78it/s]
39%|###9 | 89490/228483 [00:08<00:13, 10374.90it/s]
40%|###9 | 90534/228483 [00:09<00:13, 10391.54it/s]
40%|#### | 91574/228483 [00:09<00:13, 10347.13it/s]
41%|#### | 92618/228483 [00:09<00:13, 10373.50it/s]
41%|#### | 93657/228483 [00:09<00:12, 10376.63it/s]
41%|####1 | 94703/228483 [00:09<00:12, 10400.43it/s]
42%|####1 | 95744/228483 [00:09<00:12, 10338.09it/s]
42%|####2 | 96778/228483 [00:09<00:12, 10317.95it/s]
43%|####2 | 97822/228483 [00:09<00:12, 10352.15it/s]
43%|####3 | 98858/228483 [00:09<00:12, 10343.70it/s]
44%|####3 | 99903/228483 [00:09<00:12, 10374.50it/s]
44%|####4 | 100953/228483 [00:10<00:12, 10410.22it/s]
45%|####4 | 101995/228483 [00:10<00:12, 10347.91it/s]
45%|####5 | 103040/228483 [00:10<00:12, 10377.86it/s]
46%|####5 | 104078/228483 [00:10<00:12, 10349.94it/s]
46%|####6 | 105128/228483 [00:10<00:11, 10394.05it/s]
46%|####6 | 106168/228483 [00:10<00:11, 10323.60it/s]
47%|####6 | 107215/228483 [00:10<00:11, 10365.79it/s]
47%|####7 | 108255/228483 [00:10<00:11, 10374.91it/s]
48%|####7 | 109293/228483 [00:10<00:11, 10370.86it/s]
48%|####8 | 110338/228483 [00:10<00:11, 10392.03it/s]
49%|####8 | 111386/228483 [00:11<00:11, 10417.47it/s]
49%|####9 | 112428/228483 [00:11<00:11, 10364.35it/s]
50%|####9 | 113465/228483 [00:11<00:16, 6987.56it/s]
50%|##### | 114514/228483 [00:11<00:14, 7768.51it/s]
51%|##### | 115521/228483 [00:11<00:13, 8324.17it/s]
51%|#####1 | 116558/228483 [00:11<00:12, 8848.90it/s]
51%|#####1 | 117588/228483 [00:11<00:12, 9237.15it/s]
52%|#####1 | 118633/228483 [00:11<00:11, 9571.13it/s]
52%|#####2 | 119682/228483 [00:11<00:11, 9830.48it/s]
53%|#####2 | 120701/228483 [00:12<00:10, 9933.98it/s]
53%|#####3 | 121748/228483 [00:12<00:10, 10088.33it/s]
54%|#####3 | 122783/228483 [00:12<00:10, 10161.09it/s]
54%|#####4 | 123829/228483 [00:12<00:10, 10247.93it/s]
55%|#####4 | 124863/228483 [00:12<00:10, 10229.52it/s]
55%|#####5 | 125905/228483 [00:12<00:09, 10284.60it/s]
56%|#####5 | 126946/228483 [00:12<00:09, 10321.74it/s]
56%|#####6 | 127995/228483 [00:12<00:09, 10368.59it/s]
56%|#####6 | 129034/228483 [00:12<00:09, 10349.74it/s]
57%|#####6 | 130080/228483 [00:12<00:09, 10382.63it/s]
57%|#####7 | 131120/228483 [00:13<00:09, 10357.15it/s]
58%|#####7 | 132160/228483 [00:13<00:09, 10368.56it/s]
58%|#####8 | 133199/228483 [00:13<00:09, 10372.57it/s]
59%|#####8 | 134247/228483 [00:13<00:09, 10402.41it/s]
59%|#####9 | 135298/228483 [00:13<00:08, 10432.04it/s]
60%|#####9 | 136342/228483 [00:13<00:08, 10259.31it/s]
60%|###### | 137382/228483 [00:13<00:08, 10300.93it/s]
61%|###### | 138414/228483 [00:13<00:08, 10306.54it/s]
61%|######1 | 139463/228483 [00:13<00:08, 10360.25it/s]
61%|######1 | 140511/228483 [00:14<00:08, 10395.81it/s]
62%|######1 | 141551/228483 [00:14<00:08, 10335.32it/s]
62%|######2 | 142596/228483 [00:14<00:08, 10369.07it/s]
63%|######2 | 143634/228483 [00:14<00:08, 10368.37it/s]
63%|######3 | 144682/228483 [00:14<00:08, 10400.21it/s]
64%|######3 | 145723/228483 [00:14<00:08, 10328.93it/s]
64%|######4 | 146769/228483 [00:14<00:07, 10366.15it/s]
65%|######4 | 147810/228483 [00:14<00:07, 10376.97it/s]
65%|######5 | 148862/228483 [00:14<00:07, 10418.89it/s]
66%|######5 | 149904/228483 [00:14<00:07, 10366.70it/s]
66%|######6 | 150955/228483 [00:15<00:07, 10409.32it/s]
67%|######6 | 151997/228483 [00:15<00:07, 10363.58it/s]
67%|######6 | 153043/228483 [00:15<00:07, 10390.82it/s]
67%|######7 | 154083/228483 [00:15<00:07, 10380.51it/s]
68%|######7 | 155134/228483 [00:15<00:07, 10418.85it/s]
68%|######8 | 156176/228483 [00:15<00:06, 10411.82it/s]
69%|######8 | 157218/228483 [00:15<00:06, 10347.80it/s]
69%|######9 | 158259/228483 [00:15<00:06, 10364.75it/s]
70%|######9 | 159299/228483 [00:15<00:06, 10375.27it/s]
70%|####### | 160345/228483 [00:15<00:06, 10399.14it/s]
71%|####### | 161399/228483 [00:16<00:06, 10438.64it/s]
71%|#######1 | 162443/228483 [00:16<00:06, 10373.88it/s]
72%|#######1 | 163487/228483 [00:16<00:06, 10393.03it/s]
72%|#######2 | 164527/228483 [00:16<00:06, 10377.33it/s]
72%|#######2 | 165579/228483 [00:16<00:06, 10418.62it/s]
73%|#######2 | 166621/228483 [00:16<00:05, 10334.10it/s]
73%|#######3 | 167668/228483 [00:16<00:05, 10371.95it/s]
74%|#######3 | 168706/228483 [00:16<00:05, 10373.71it/s]
74%|#######4 | 169757/228483 [00:16<00:05, 10413.94it/s]
75%|#######4 | 170799/228483 [00:16<00:05, 10372.12it/s]
75%|#######5 | 171837/228483 [00:17<00:08, 6754.56it/s]
76%|#######5 | 172869/228483 [00:17<00:07, 7529.14it/s]
76%|#######6 | 173919/228483 [00:17<00:06, 8231.90it/s]
77%|#######6 | 174963/228483 [00:17<00:06, 8789.51it/s]
77%|#######7 | 175973/228483 [00:17<00:05, 9136.02it/s]
77%|#######7 | 177008/228483 [00:17<00:05, 9468.63it/s]
78%|#######7 | 178007/228483 [00:17<00:05, 9355.32it/s]
78%|#######8 | 179050/228483 [00:17<00:05, 9657.16it/s]
79%|#######8 | 180094/228483 [00:18<00:04, 9880.09it/s]
79%|#######9 | 181117/228483 [00:18<00:04, 9980.12it/s]
80%|#######9 | 182156/228483 [00:18<00:04, 10098.60it/s]
80%|######## | 183194/228483 [00:18<00:04, 10179.60it/s]
81%|######## | 184241/228483 [00:18<00:04, 10264.42it/s]
81%|########1 | 185273/228483 [00:18<00:04, 10246.89it/s]
82%|########1 | 186315/228483 [00:18<00:04, 10297.93it/s]
82%|########2 | 187358/228483 [00:18<00:03, 10335.46it/s]
82%|########2 | 188406/228483 [00:18<00:03, 10378.10it/s]
83%|########2 | 189446/228483 [00:18<00:03, 10362.04it/s]
83%|########3 | 190494/228483 [00:19<00:03, 10395.19it/s]
84%|########3 | 191535/228483 [00:19<00:03, 10358.41it/s]
84%|########4 | 192575/228483 [00:19<00:03, 10369.62it/s]
85%|########4 | 193615/228483 [00:19<00:03, 10368.73it/s]
85%|########5 | 194665/228483 [00:19<00:03, 10407.14it/s]
86%|########5 | 195709/228483 [00:19<00:03, 10416.16it/s]
86%|########6 | 196751/228483 [00:19<00:03, 10336.95it/s]
87%|########6 | 197790/228483 [00:19<00:02, 10350.90it/s]
87%|########7 | 198829/228483 [00:19<00:02, 10361.47it/s]
87%|########7 | 199873/228483 [00:19<00:02, 10383.25it/s]
88%|########7 | 200924/228483 [00:20<00:02, 10420.25it/s]
88%|########8 | 201967/228483 [00:20<00:02, 10355.85it/s]
89%|########8 | 203011/228483 [00:20<00:02, 10378.58it/s]
89%|########9 | 204049/228483 [00:20<00:02, 10366.27it/s]
90%|########9 | 205101/228483 [00:20<00:02, 10411.98it/s]
90%|######### | 206143/228483 [00:20<00:02, 10357.54it/s]
91%|######### | 207190/228483 [00:20<00:02, 10390.36it/s]
91%|#########1| 208230/228483 [00:20<00:01, 10380.15it/s]
92%|#########1| 209280/228483 [00:20<00:01, 10414.29it/s]
92%|#########2| 210322/228483 [00:20<00:01, 10382.13it/s]
93%|#########2| 211374/228483 [00:21<00:01, 10421.29it/s]
93%|#########2| 212417/228483 [00:21<00:01, 10370.70it/s]
93%|#########3| 213466/228483 [00:21<00:01, 10404.38it/s]
94%|#########3| 214507/228483 [00:21<00:01, 10401.35it/s]
94%|#########4| 215561/228483 [00:21<00:01, 10442.20it/s]
95%|#########4| 216606/228483 [00:21<00:01, 10408.86it/s]
95%|#########5| 217647/228483 [00:21<00:01, 10346.75it/s]
96%|#########5| 218692/228483 [00:21<00:00, 10377.12it/s]
96%|#########6| 219730/228483 [00:21<00:00, 10374.47it/s]
97%|#########6| 220773/228483 [00:21<00:00, 10390.69it/s]
97%|#########7| 221823/228483 [00:22<00:00, 10422.90it/s]
98%|#########7| 222866/228483 [00:22<00:00, 10368.96it/s]
98%|#########8| 223915/228483 [00:22<00:00, 10403.41it/s]
98%|#########8| 224956/228483 [00:22<00:00, 10383.87it/s]
99%|#########8| 226009/228483 [00:22<00:00, 10427.22it/s]
99%|#########9| 227052/228483 [00:22<00:00, 10354.56it/s]
100%|#########9| 228101/228483 [00:22<00:00, 10392.10it/s]
100%|##########| 228483/228483 [00:29<00:00, 7702.73it/s]
<nilearn.plotting.displays._slicers.OrthoSlicer object at 0x7f1848a9fb10>
Total running time of the script: ( 1 minutes 14.293 seconds)