Note
Click here to download the full example code
Coordinate-based meta-analysis algorithms
A tour of CBMA algorithms in NiMARE.
This tutorial is intended to provide a brief description and example of each of the CBMA algorithms implemented in NiMARE. For a more detailed introduction to the elements of a coordinate-based meta-analysis, see other stuff.
Load Dataset
Note
The data used in this example come from a collection of NIDM-Results packs downloaded from Neurovault collection 1425, uploaded by Dr. Camille Maumet.
Creation of the Dataset from the NIDM-Results packs was done with custom code. The Results packs for collection 1425 are not completely NIDM-Results-compliant, so the nidmresults library could not be used to facilitate data extraction.
import os
from nilearn.plotting import plot_stat_map
from nimare.correct import FWECorrector
from nimare.dataset import Dataset
from nimare.utils import get_resource_path
dset_file = os.path.join(get_resource_path(), "nidm_pain_dset.json")
dset = Dataset(dset_file)
# Some of the CBMA algorithms compare two Datasets,
# so we'll split this example Dataset in half.
dset1 = dset.slice(dset.ids[:10])
dset2 = dset.slice(dset.ids[10:])
Multilevel Kernel Density Analysis
from nimare.meta.cbma.mkda import MKDADensity
meta = MKDADensity()
results = meta.fit(dset)
corr = FWECorrector(method="montecarlo", n_iters=10, n_cores=1)
cres = corr.transform(results)
plot_stat_map(
results.get_map("z"),
cut_coords=[0, 0, -8],
draw_cross=False,
cmap="RdBu_r",
threshold=0.1,
)
plot_stat_map(
cres.get_map("z_level-voxel_corr-FWE_method-montecarlo"),
cut_coords=[0, 0, -8],
draw_cross=False,
cmap="RdBu_r",
threshold=0.1,
)
Out:
0%| | 0/10 [00:00<?, ?it/s]
10%|# | 1/10 [00:00<00:03, 2.64it/s]
20%|## | 2/10 [00:00<00:02, 2.67it/s]
30%|### | 3/10 [00:01<00:02, 2.72it/s]
40%|#### | 4/10 [00:01<00:02, 2.74it/s]
50%|##### | 5/10 [00:01<00:01, 2.76it/s]
60%|###### | 6/10 [00:02<00:01, 2.73it/s]
70%|####### | 7/10 [00:02<00:01, 2.72it/s]
80%|######## | 8/10 [00:02<00:00, 2.73it/s]
90%|######### | 9/10 [00:03<00:00, 2.73it/s]
100%|##########| 10/10 [00:03<00:00, 2.74it/s]
100%|##########| 10/10 [00:03<00:00, 2.73it/s]
<nilearn.plotting.displays._slicers.OrthoSlicer object at 0x7fe0c357ca90>
MKDA Chi-Squared
from nimare.meta.cbma.mkda import MKDAChi2
meta = MKDAChi2(kernel__r=10)
results = meta.fit(dset1, dset2)
corr = FWECorrector(method="montecarlo", n_iters=10, n_cores=1)
cres = corr.transform(results)
plot_stat_map(
results.get_map("z_desc-consistency"),
draw_cross=False,
cmap="RdBu_r",
threshold=0.1,
)
plot_stat_map(
cres.get_map("z_desc-consistencySize_level-cluster_corr-FWE_method-montecarlo"),
draw_cross=False,
cmap="RdBu_r",
threshold=0.1,
)
Out:
/home/docs/checkouts/readthedocs.org/user_builds/nimare/checkouts/0.0.12/nimare/meta/cbma/mkda.py:396: RuntimeWarning: invalid value encountered in true_divide
pFgA = pAgF * pF / pA
/home/docs/checkouts/readthedocs.org/user_builds/nimare/checkouts/0.0.12/nimare/meta/cbma/mkda.py:402: RuntimeWarning: invalid value encountered in true_divide
pFgA_prior = pAgF * self.prior / pAgF_prior
0%| | 0/10 [00:00<?, ?it/s]
10%|# | 1/10 [00:00<00:06, 1.40it/s]
20%|## | 2/10 [00:01<00:05, 1.46it/s]
30%|### | 3/10 [00:02<00:04, 1.47it/s]
40%|#### | 4/10 [00:02<00:04, 1.48it/s]
50%|##### | 5/10 [00:03<00:03, 1.48it/s]
60%|###### | 6/10 [00:04<00:02, 1.47it/s]
70%|####### | 7/10 [00:04<00:02, 1.47it/s]
80%|######## | 8/10 [00:05<00:01, 1.48it/s]
90%|######### | 9/10 [00:06<00:00, 1.48it/s]
100%|##########| 10/10 [00:06<00:00, 1.47it/s]
100%|##########| 10/10 [00:06<00:00, 1.47it/s]
<nilearn.plotting.displays._slicers.OrthoSlicer object at 0x7fe0c35a1f10>
Kernel Density Analysis
from nimare.meta.cbma.mkda import KDA
meta = KDA()
results = meta.fit(dset)
corr = FWECorrector(method="montecarlo", n_iters=10, n_cores=1)
cres = corr.transform(results)
plot_stat_map(
results.get_map("z"),
cut_coords=[0, 0, -8],
draw_cross=False,
cmap="RdBu_r",
threshold=0.1,
)
plot_stat_map(
cres.get_map("z_desc-size_level-cluster_corr-FWE_method-montecarlo"),
cut_coords=[0, 0, -8],
draw_cross=False,
cmap="RdBu_r",
threshold=0.1,
)
Out:
0%| | 0/10 [00:00<?, ?it/s]
10%|# | 1/10 [00:00<00:03, 2.52it/s]
20%|## | 2/10 [00:00<00:03, 2.52it/s]
30%|### | 3/10 [00:01<00:02, 2.54it/s]
40%|#### | 4/10 [00:01<00:02, 2.53it/s]
50%|##### | 5/10 [00:01<00:01, 2.55it/s]
60%|###### | 6/10 [00:02<00:01, 2.55it/s]
70%|####### | 7/10 [00:02<00:01, 2.53it/s]
80%|######## | 8/10 [00:03<00:00, 2.54it/s]
90%|######### | 9/10 [00:03<00:00, 2.52it/s]
100%|##########| 10/10 [00:03<00:00, 2.54it/s]
100%|##########| 10/10 [00:03<00:00, 2.54it/s]
<nilearn.plotting.displays._slicers.OrthoSlicer object at 0x7fe0c34a0a10>
Activation Likelihood Estimation
from nimare.meta.cbma.ale import ALE
meta = ALE()
results = meta.fit(dset)
corr = FWECorrector(method="montecarlo", n_iters=10, n_cores=1)
cres = corr.transform(results)
plot_stat_map(
results.get_map("z"),
cut_coords=[0, 0, -8],
draw_cross=False,
cmap="RdBu_r",
threshold=0.1,
)
plot_stat_map(
cres.get_map("z_desc-size_level-cluster_corr-FWE_method-montecarlo"),
cut_coords=[0, 0, -8],
draw_cross=False,
cmap="RdBu_r",
threshold=0.1,
)
Out:
0%| | 0/10 [00:00<?, ?it/s]
10%|# | 1/10 [00:00<00:05, 1.58it/s]
20%|## | 2/10 [00:01<00:05, 1.60it/s]
30%|### | 3/10 [00:01<00:04, 1.61it/s]
40%|#### | 4/10 [00:02<00:03, 1.61it/s]
50%|##### | 5/10 [00:03<00:03, 1.62it/s]
60%|###### | 6/10 [00:03<00:02, 1.61it/s]
70%|####### | 7/10 [00:04<00:01, 1.62it/s]
80%|######## | 8/10 [00:04<00:01, 1.63it/s]
90%|######### | 9/10 [00:05<00:00, 1.62it/s]
100%|##########| 10/10 [00:06<00:00, 1.63it/s]
100%|##########| 10/10 [00:06<00:00, 1.62it/s]
<nilearn.plotting.displays._slicers.OrthoSlicer object at 0x7fe0c3669290>
Specific Co-Activation Likelihood Estimation
Important
The SCALE algorithm is very memory intensive, so we don’t run it within the documentation.
ALE-Based Subtraction Analysis
from nimare.meta.cbma.ale import ALESubtraction
meta = ALESubtraction(n_iters=10, n_cores=1)
results = meta.fit(dset1, dset2)
plot_stat_map(
results.get_map("z_desc-group1MinusGroup2"),
cut_coords=[0, 0, -8],
draw_cross=False,
cmap="RdBu_r",
threshold=0.1,
)
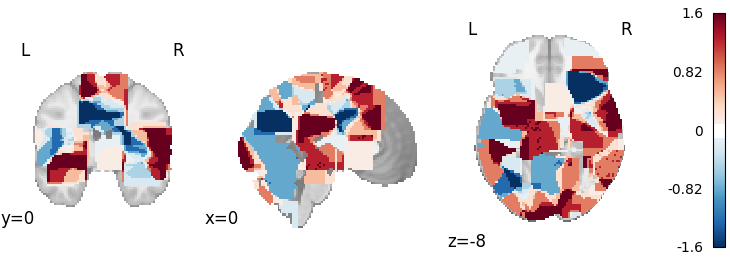
Out:
0%| | 0/10 [00:00<?, ?it/s]
10%|# | 1/10 [00:03<00:27, 3.11s/it]
20%|## | 2/10 [00:03<00:11, 1.41s/it]
30%|### | 3/10 [00:03<00:06, 1.15it/s]
40%|#### | 4/10 [00:03<00:03, 1.62it/s]
50%|##### | 5/10 [00:04<00:02, 2.11it/s]
60%|###### | 6/10 [00:04<00:01, 2.57it/s]
70%|####### | 7/10 [00:04<00:01, 2.98it/s]
80%|######## | 8/10 [00:04<00:00, 3.32it/s]
90%|######### | 9/10 [00:04<00:00, 3.60it/s]
100%|##########| 10/10 [00:05<00:00, 3.80it/s]
100%|##########| 10/10 [00:05<00:00, 1.95it/s]
0%| | 0/228483 [00:00<?, ?it/s]
0%| | 1041/228483 [00:00<00:21, 10400.69it/s]
1%| | 2082/228483 [00:00<00:21, 10316.04it/s]
1%|1 | 3132/228483 [00:00<00:21, 10396.05it/s]
2%|1 | 4172/228483 [00:00<00:21, 10384.93it/s]
2%|2 | 5224/228483 [00:00<00:21, 10430.28it/s]
3%|2 | 6270/228483 [00:00<00:21, 10438.71it/s]
3%|3 | 7314/228483 [00:00<00:21, 10435.66it/s]
4%|3 | 8358/228483 [00:00<00:21, 10428.06it/s]
4%|4 | 9401/228483 [00:00<00:21, 10423.68it/s]
5%|4 | 10444/228483 [00:01<00:20, 10424.25it/s]
5%|5 | 11489/228483 [00:01<00:20, 10431.13it/s]
5%|5 | 12541/228483 [00:01<00:20, 10456.00it/s]
6%|5 | 13592/228483 [00:01<00:20, 10470.38it/s]
6%|6 | 14640/228483 [00:01<00:20, 10457.00it/s]
7%|6 | 15687/228483 [00:01<00:20, 10459.26it/s]
7%|7 | 16739/228483 [00:01<00:20, 10476.48it/s]
8%|7 | 17787/228483 [00:01<00:20, 10471.25it/s]
8%|8 | 18839/228483 [00:01<00:19, 10484.94it/s]
9%|8 | 19888/228483 [00:01<00:19, 10474.77it/s]
9%|9 | 20936/228483 [00:02<00:19, 10412.11it/s]
10%|9 | 21978/228483 [00:02<00:19, 10407.88it/s]
10%|# | 23019/228483 [00:02<00:28, 7308.50it/s]
11%|# | 24063/228483 [00:02<00:25, 8029.21it/s]
11%|# | 25110/228483 [00:02<00:23, 8633.31it/s]
11%|#1 | 26170/228483 [00:02<00:22, 9145.43it/s]
12%|#1 | 27214/228483 [00:02<00:21, 9496.42it/s]
12%|#2 | 28254/228483 [00:02<00:20, 9748.09it/s]
13%|#2 | 29296/228483 [00:02<00:20, 9939.50it/s]
13%|#3 | 30342/228483 [00:03<00:19, 10087.98it/s]
14%|#3 | 31390/228483 [00:03<00:19, 10202.41it/s]
14%|#4 | 32436/228483 [00:03<00:19, 10276.48it/s]
15%|#4 | 33485/228483 [00:03<00:18, 10339.02it/s]
15%|#5 | 34532/228483 [00:03<00:18, 10376.05it/s]
16%|#5 | 35575/228483 [00:03<00:18, 10387.76it/s]
16%|#6 | 36622/228483 [00:03<00:18, 10410.56it/s]
16%|#6 | 37670/228483 [00:03<00:18, 10430.89it/s]
17%|#6 | 38717/228483 [00:03<00:18, 10440.74it/s]
17%|#7 | 39763/228483 [00:03<00:18, 10434.82it/s]
18%|#7 | 40808/228483 [00:04<00:18, 10418.56it/s]
18%|#8 | 41851/228483 [00:04<00:17, 10407.03it/s]
19%|#8 | 42893/228483 [00:04<00:17, 10391.55it/s]
19%|#9 | 43937/228483 [00:04<00:17, 10405.53it/s]
20%|#9 | 44978/228483 [00:04<00:17, 10394.50it/s]
20%|## | 46024/228483 [00:04<00:17, 10411.53it/s]
21%|## | 47078/228483 [00:04<00:17, 10448.36it/s]
21%|##1 | 48123/228483 [00:04<00:17, 10432.81it/s]
22%|##1 | 49167/228483 [00:04<00:17, 10400.66it/s]
22%|##1 | 50208/228483 [00:04<00:17, 10396.28it/s]
22%|##2 | 51250/228483 [00:05<00:17, 10403.27it/s]
23%|##2 | 52301/228483 [00:05<00:16, 10433.06it/s]
23%|##3 | 53349/228483 [00:05<00:16, 10446.98it/s]
24%|##3 | 54401/228483 [00:05<00:16, 10466.73it/s]
24%|##4 | 55448/228483 [00:05<00:16, 10447.75it/s]
25%|##4 | 56497/228483 [00:05<00:16, 10458.49it/s]
25%|##5 | 57543/228483 [00:05<00:16, 10455.02it/s]
26%|##5 | 58589/228483 [00:05<00:23, 7324.51it/s]
26%|##6 | 59618/228483 [00:05<00:21, 8007.36it/s]
27%|##6 | 60652/228483 [00:06<00:19, 8585.27it/s]
27%|##6 | 61678/228483 [00:06<00:18, 9021.75it/s]
27%|##7 | 62722/228483 [00:06<00:17, 9406.72it/s]
28%|##7 | 63762/228483 [00:06<00:17, 9684.15it/s]
28%|##8 | 64795/228483 [00:06<00:16, 9866.67it/s]
29%|##8 | 65843/228483 [00:06<00:16, 10042.99it/s]
29%|##9 | 66879/228483 [00:06<00:15, 10133.65it/s]
30%|##9 | 67927/228483 [00:06<00:15, 10233.45it/s]
30%|### | 68961/228483 [00:06<00:15, 10232.05it/s]
31%|### | 70005/228483 [00:06<00:15, 10293.67it/s]
31%|###1 | 71040/228483 [00:07<00:15, 10309.88it/s]
32%|###1 | 72090/228483 [00:07<00:15, 10364.13it/s]
32%|###2 | 73129/228483 [00:07<00:14, 10367.58it/s]
32%|###2 | 74171/228483 [00:07<00:14, 10380.52it/s]
33%|###2 | 75211/228483 [00:07<00:14, 10383.66it/s]
33%|###3 | 76264/228483 [00:07<00:14, 10425.12it/s]
34%|###3 | 77308/228483 [00:07<00:14, 10418.41it/s]
34%|###4 | 78351/228483 [00:07<00:14, 10345.65it/s]
35%|###4 | 79389/228483 [00:07<00:14, 10354.29it/s]
35%|###5 | 80425/228483 [00:08<00:14, 10339.34it/s]
36%|###5 | 81462/228483 [00:08<00:14, 10347.71it/s]
36%|###6 | 82497/228483 [00:08<00:14, 10334.95it/s]
37%|###6 | 83542/228483 [00:08<00:13, 10369.22it/s]
37%|###7 | 84580/228483 [00:08<00:13, 10362.84it/s]
37%|###7 | 85619/228483 [00:08<00:13, 10369.02it/s]
38%|###7 | 86664/228483 [00:08<00:13, 10391.19it/s]
38%|###8 | 87704/228483 [00:08<00:13, 10380.31it/s]
39%|###8 | 88757/228483 [00:08<00:13, 10423.50it/s]
39%|###9 | 89800/228483 [00:08<00:13, 10361.75it/s]
40%|###9 | 90844/228483 [00:09<00:13, 10383.92it/s]
40%|#### | 91883/228483 [00:09<00:13, 10370.40it/s]
41%|#### | 92934/228483 [00:09<00:13, 10411.94it/s]
41%|####1 | 93976/228483 [00:09<00:12, 10407.25it/s]
42%|####1 | 95017/228483 [00:09<00:12, 10281.71it/s]
42%|####2 | 96054/228483 [00:09<00:12, 10305.41it/s]
42%|####2 | 97104/228483 [00:09<00:12, 10361.13it/s]
43%|####2 | 98146/228483 [00:09<00:12, 10376.74it/s]
43%|####3 | 99195/228483 [00:09<00:12, 10409.74it/s]
44%|####3 | 100237/228483 [00:09<00:12, 10402.61it/s]
44%|####4 | 101278/228483 [00:10<00:12, 10377.38it/s]
45%|####4 | 102316/228483 [00:10<00:18, 7008.62it/s]
45%|####5 | 103353/228483 [00:10<00:16, 7759.93it/s]
46%|####5 | 104386/228483 [00:10<00:14, 8382.10it/s]
46%|####6 | 105431/228483 [00:10<00:13, 8913.29it/s]
47%|####6 | 106478/228483 [00:10<00:13, 9329.29it/s]
47%|####7 | 107525/228483 [00:10<00:12, 9644.38it/s]
48%|####7 | 108552/228483 [00:10<00:12, 9820.93it/s]
48%|####7 | 109594/228483 [00:10<00:11, 9993.10it/s]
48%|####8 | 110633/228483 [00:11<00:11, 10107.40it/s]
49%|####8 | 111688/228483 [00:11<00:11, 10236.70it/s]
49%|####9 | 112725/228483 [00:11<00:11, 10275.50it/s]
50%|####9 | 113778/228483 [00:11<00:11, 10349.09it/s]
50%|##### | 114819/228483 [00:11<00:10, 10345.98it/s]
51%|##### | 115872/228483 [00:11<00:10, 10400.80it/s]
51%|#####1 | 116916/228483 [00:11<00:10, 10394.96it/s]
52%|#####1 | 117968/228483 [00:11<00:10, 10431.99it/s]
52%|#####2 | 119013/228483 [00:11<00:10, 10421.83it/s]
53%|#####2 | 120057/228483 [00:11<00:10, 10394.15it/s]
53%|#####3 | 121098/228483 [00:12<00:10, 10395.61it/s]
53%|#####3 | 122144/228483 [00:12<00:10, 10412.66it/s]
54%|#####3 | 123186/228483 [00:12<00:10, 10387.32it/s]
54%|#####4 | 124231/228483 [00:12<00:10, 10405.70it/s]
55%|#####4 | 125272/228483 [00:12<00:09, 10382.36it/s]
55%|#####5 | 126322/228483 [00:12<00:09, 10415.60it/s]
56%|#####5 | 127365/228483 [00:12<00:09, 10417.61it/s]
56%|#####6 | 128419/228483 [00:12<00:09, 10453.14it/s]
57%|#####6 | 129465/228483 [00:12<00:09, 10400.44it/s]
57%|#####7 | 130509/228483 [00:12<00:09, 10410.49it/s]
58%|#####7 | 131551/228483 [00:13<00:09, 10396.95it/s]
58%|#####8 | 132599/228483 [00:13<00:09, 10421.14it/s]
58%|#####8 | 133642/228483 [00:13<00:09, 10404.24it/s]
59%|#####8 | 134688/228483 [00:13<00:09, 10418.88it/s]
59%|#####9 | 135730/228483 [00:13<00:08, 10418.06it/s]
60%|#####9 | 136784/228483 [00:13<00:08, 10453.40it/s]
60%|###### | 137830/228483 [00:13<00:08, 10441.80it/s]
61%|###### | 138884/228483 [00:13<00:08, 10470.48it/s]
61%|######1 | 139932/228483 [00:13<00:08, 10455.56it/s]
62%|######1 | 140978/228483 [00:13<00:08, 10417.62it/s]
62%|######2 | 142020/228483 [00:14<00:08, 10416.59it/s]
63%|######2 | 143066/228483 [00:14<00:08, 10428.62it/s]
63%|######3 | 144109/228483 [00:14<00:08, 10393.78it/s]
64%|######3 | 145155/228483 [00:14<00:08, 10412.37it/s]
64%|######3 | 146197/228483 [00:14<00:07, 10377.70it/s]
64%|######4 | 147247/228483 [00:14<00:07, 10411.50it/s]
65%|######4 | 148289/228483 [00:14<00:07, 10405.20it/s]
65%|######5 | 149340/228483 [00:14<00:07, 10435.47it/s]
66%|######5 | 150384/228483 [00:14<00:07, 10377.61it/s]
66%|######6 | 151432/228483 [00:14<00:07, 10405.43it/s]
67%|######6 | 152473/228483 [00:15<00:07, 10393.87it/s]
67%|######7 | 153526/228483 [00:15<00:07, 10432.71it/s]
68%|######7 | 154570/228483 [00:15<00:07, 10418.97it/s]
68%|######8 | 155617/228483 [00:15<00:06, 10431.39it/s]
69%|######8 | 156661/228483 [00:15<00:10, 6830.23it/s]
69%|######9 | 157712/228483 [00:15<00:09, 7634.34it/s]
69%|######9 | 158751/228483 [00:15<00:08, 8288.80it/s]
70%|######9 | 159756/228483 [00:15<00:07, 8733.80it/s]
70%|####### | 160791/228483 [00:16<00:07, 9162.14it/s]
71%|####### | 161835/228483 [00:16<00:07, 9513.06it/s]
71%|#######1 | 162863/228483 [00:16<00:06, 9728.04it/s]
72%|#######1 | 163909/228483 [00:16<00:06, 9937.15it/s]
72%|#######2 | 164942/228483 [00:16<00:06, 10049.86it/s]
73%|#######2 | 165991/228483 [00:16<00:06, 10176.47it/s]
73%|#######3 | 167037/228483 [00:16<00:05, 10259.77it/s]
74%|#######3 | 168078/228483 [00:16<00:05, 10301.76it/s]
74%|#######4 | 169115/228483 [00:16<00:05, 10292.44it/s]
74%|#######4 | 170158/228483 [00:16<00:05, 10330.78it/s]
75%|#######4 | 171195/228483 [00:17<00:05, 10341.60it/s]
75%|#######5 | 172245/228483 [00:17<00:05, 10388.80it/s]
76%|#######5 | 173288/228483 [00:17<00:05, 10399.31it/s]
76%|#######6 | 174343/228483 [00:17<00:05, 10444.08it/s]
77%|#######6 | 175389/228483 [00:17<00:05, 10416.45it/s]
77%|#######7 | 176443/228483 [00:17<00:04, 10450.64it/s]
78%|#######7 | 177489/228483 [00:17<00:04, 10439.19it/s]
78%|#######8 | 178541/228483 [00:17<00:04, 10463.22it/s]
79%|#######8 | 179588/228483 [00:17<00:04, 10449.73it/s]
79%|#######9 | 180634/228483 [00:17<00:04, 10426.04it/s]
80%|#######9 | 181677/228483 [00:18<00:04, 10278.37it/s]
80%|#######9 | 182723/228483 [00:18<00:04, 10331.14it/s]
80%|######## | 183757/228483 [00:18<00:04, 10317.68it/s]
81%|######## | 184803/228483 [00:18<00:04, 10358.43it/s]
81%|########1 | 185840/228483 [00:18<00:04, 10346.46it/s]
82%|########1 | 186889/228483 [00:18<00:04, 10387.03it/s]
82%|########2 | 187937/228483 [00:18<00:03, 10413.46it/s]
83%|########2 | 188979/228483 [00:18<00:03, 10410.79it/s]
83%|########3 | 190021/228483 [00:18<00:03, 10369.20it/s]
84%|########3 | 191063/228483 [00:18<00:03, 10382.80it/s]
84%|########4 | 192102/228483 [00:19<00:03, 10379.60it/s]
85%|########4 | 193156/228483 [00:19<00:03, 10425.73it/s]
85%|########4 | 194199/228483 [00:19<00:03, 10413.83it/s]
85%|########5 | 195250/228483 [00:19<00:03, 10440.21it/s]
86%|########5 | 196295/228483 [00:19<00:03, 10411.96it/s]
86%|########6 | 197348/228483 [00:19<00:02, 10444.98it/s]
87%|########6 | 198393/228483 [00:19<00:02, 10436.04it/s]
87%|########7 | 199447/228483 [00:19<00:02, 10464.93it/s]
88%|########7 | 200494/228483 [00:19<00:02, 10448.29it/s]
88%|########8 | 201539/228483 [00:19<00:02, 10413.92it/s]
89%|########8 | 202581/228483 [00:20<00:02, 10410.16it/s]
89%|########9 | 203629/228483 [00:20<00:02, 10428.37it/s]
90%|########9 | 204672/228483 [00:20<00:02, 10393.78it/s]
90%|######### | 205717/228483 [00:20<00:02, 10409.48it/s]
90%|######### | 206758/228483 [00:20<00:02, 10379.85it/s]
91%|######### | 207806/228483 [00:20<00:01, 10408.28it/s]
91%|#########1| 208853/228483 [00:20<00:01, 10426.58it/s]
92%|#########1| 209897/228483 [00:20<00:01, 10429.49it/s]
92%|#########2| 210940/228483 [00:20<00:01, 10381.22it/s]
93%|#########2| 211979/228483 [00:20<00:01, 10344.11it/s]
93%|#########3| 213020/228483 [00:21<00:01, 10362.96it/s]
94%|#########3| 214075/228483 [00:21<00:01, 10418.63it/s]
94%|#########4| 215122/228483 [00:21<00:01, 10431.36it/s]
95%|#########4| 216178/228483 [00:21<00:01, 10467.22it/s]
95%|#########5| 217225/228483 [00:21<00:01, 10441.34it/s]
96%|#########5| 218279/228483 [00:21<00:00, 10468.76it/s]
96%|#########5| 219326/228483 [00:21<00:00, 10453.08it/s]
96%|#########6| 220379/228483 [00:21<00:00, 10475.52it/s]
97%|#########6| 221427/228483 [00:21<00:00, 10450.74it/s]
97%|#########7| 222473/228483 [00:21<00:00, 10415.81it/s]
98%|#########7| 223516/228483 [00:22<00:00, 10417.98it/s]
98%|#########8| 224564/228483 [00:22<00:00, 10423.46it/s]
99%|#########8| 225607/228483 [00:22<00:00, 6440.03it/s]
99%|#########9| 226654/228483 [00:22<00:00, 7280.02it/s]
100%|#########9| 227688/228483 [00:22<00:00, 7981.15it/s]
100%|##########| 228483/228483 [00:29<00:00, 7760.59it/s]
<nilearn.plotting.displays._slicers.OrthoSlicer object at 0x7fe0c3284710>
Total running time of the script: ( 1 minutes 20.208 seconds)